This is a long time i have never had a post. Today, i will introduce you how to convert int and long long number to std::string.
When i join in a project about fuzzing browsers, i have to use a very large data type because i must count the number of requests to browsers and this course is continuous and it occures in a very and very long time.
So i think about long long data format. But when you send information to a browser, you must send a string request and if so, you need to convert long long to string before you send it.
When i got this problem, i found it on the internet in many famous websites, and the more websites i flurted, the more information i got. After that, i synthesized all information that i had and my work is well done.
In this topic i will introduce mainly about long long to std::string because "int" is a very popular data type.
"long long" is a data type that is used in C++, it is 64bits in length. it's range in signed is from (-2^63) to (2^63 - 1) and in unsigned is from 0 to 2^64 - 1, so you can see it is a very and very range. This is the reason that i choose it for my project.
And now, i will introduce to convert this data type to std::string
+ Convert int to std::string
std::string IntToString(int number)
{
std::stringstream out;
out << number;
return out.str();
}
+ Convert long long to std::string
std::string LongLongToString(unsigned long long number)
{
std::stringstream out;
out << number;
return out.str();
}
To use two function, you need to declare some librabry, such as: <string> and <sstream>
I hope this information will help you in your work.
Thanks for visiting my blog.
Do your best, the rest will come!
Thứ Năm, 10 tháng 11, 2011
Thứ Năm, 21 tháng 7, 2011
Relax corner
Come back to me - David Cook
You say that you're becoming someone else
Don't recognize the face in the mirror
Looking back at you
You say you're leavin
As you look away
I know theres really nothin left to say
Just know i'm here
Whenever you need me
I'll wait for you
So i'll let you go
I'll set you free
And when you see what you need to see
When you find you come back to me
Take your time i wont go anywhere
Picture you with the wind in your hair
I'll keep your things right where you left them
I'll be here for you
Oh and i'll let you go
I'll set you free
And when you see what you need to see
When you find you come back to me
And i hope you find everything that you need
I'll be right here waiting to see
You find you come back to me
I can't get close if your not there
I can't get inside if theres no soul to bear
I can't fix you i can't save you
Its something you have to do
So i'll let you go
I'll set you free
And when you see what you need to see
When you find you come back to me
Come back to me
So i'll let you go
I'll set you free
And when you see what you need to see
When you find you come back to me
And i hope you find everything that you need
I'll be right here waiting to see
You find you come back to me
When you find you come back to me
When you find you come back to me
When you find you come back to me
Lyric:
You say you gotta go and find yourselfYou say that you're becoming someone else
Don't recognize the face in the mirror
Looking back at you
You say you're leavin
As you look away
I know theres really nothin left to say
Just know i'm here
Whenever you need me
I'll wait for you
So i'll let you go
I'll set you free
And when you see what you need to see
When you find you come back to me
Take your time i wont go anywhere
Picture you with the wind in your hair
I'll keep your things right where you left them
I'll be here for you
Oh and i'll let you go
I'll set you free
And when you see what you need to see
When you find you come back to me
And i hope you find everything that you need
I'll be right here waiting to see
You find you come back to me
I can't get close if your not there
I can't get inside if theres no soul to bear
I can't fix you i can't save you
Its something you have to do
So i'll let you go
I'll set you free
And when you see what you need to see
When you find you come back to me
Come back to me
So i'll let you go
I'll set you free
And when you see what you need to see
When you find you come back to me
And i hope you find everything that you need
I'll be right here waiting to see
You find you come back to me
When you find you come back to me
When you find you come back to me
When you find you come back to me
How can i browse a folder C++, MFC
I have just finished a project and my project has got an method which allow you create a button for browse an folder. I have created a class to do this duty.
// This is getFolderPath.h
#include "shlobj.h"
#include <string>
class getFolderPath
{
public:
getFolderPath(void);
~getFolderPath(void);
static bool GetFolderPath(std::string& folderpath, HWND);
};
// This is getFolderPath.cpp
// You have to add "stdafx.h" library if you make your project in Visual Stdio
#include "getFolderPath.h"
getFolderPath::getFolderPath(void)
{
/*hOwner = hWnd;*/
}
getFolderPath::~getFolderPath(void)
{
}
bool getFolderPath::GetFolderPath(std::string& folderpath,HWND hOwner)
{
bool retVal = false;
// The BROWSEINFO struct tells the shell
// how it should display the dialog.
BROWSEINFO bi;
memset(&bi, 0, sizeof(bi));
bi.ulFlags = BIF_USENEWUI;
bi.hwndOwner = hOwner;
bi.lpszTitle = NULL;
// must call this if using BIF_USENEWUI
::OleInitialize(NULL);
// Show the dialog and get the itemIDList for the selected folder.
LPITEMIDLIST pIDL = ::SHBrowseForFolder(&bi);
if(pIDL != NULL)
{
// Create a buffer to store the path, then get the path.
char buffer[_MAX_PATH] = {L'\0'};
if(::SHGetPathFromIDListA(pIDL, buffer) != 0)
{
// Set the string value.
folderpath = buffer;
retVal = true;
}
// free the item id list
CoTaskMemFree(pIDL);
}
::OleUninitialize();
return retVal;
}
How you can use my class.
- If it is a window application or a MFC application, you have to use it's HWND for GetFolderPath function. You can get it's HWND by this function: this->GetSafeHWND();
#include "getPathFolder.h"
#include <string>
int main()
{
std::string sPath = "";
if(getPathFoder::GetFolderPath(sPath,NULL))
{
printf("You have just chosen this folder: %s",sPath.c_str());
}
else
{
// Process when can get folder's path
}
return 1;
}
// This is getFolderPath.h
#include "shlobj.h"
#include <string>
class getFolderPath
{
public:
getFolderPath(void);
~getFolderPath(void);
static bool GetFolderPath(std::string& folderpath, HWND);
};
// This is getFolderPath.cpp
// You have to add "stdafx.h" library if you make your project in Visual Stdio
#include "getFolderPath.h"
getFolderPath::getFolderPath(void)
{
/*hOwner = hWnd;*/
}
getFolderPath::~getFolderPath(void)
{
}
bool getFolderPath::GetFolderPath(std::string& folderpath,HWND hOwner)
{
bool retVal = false;
// The BROWSEINFO struct tells the shell
// how it should display the dialog.
BROWSEINFO bi;
memset(&bi, 0, sizeof(bi));
bi.ulFlags = BIF_USENEWUI;
bi.hwndOwner = hOwner;
bi.lpszTitle = NULL;
// must call this if using BIF_USENEWUI
::OleInitialize(NULL);
// Show the dialog and get the itemIDList for the selected folder.
LPITEMIDLIST pIDL = ::SHBrowseForFolder(&bi);
if(pIDL != NULL)
{
// Create a buffer to store the path, then get the path.
char buffer[_MAX_PATH] = {L'\0'};
if(::SHGetPathFromIDListA(pIDL, buffer) != 0)
{
// Set the string value.
folderpath = buffer;
retVal = true;
}
// free the item id list
CoTaskMemFree(pIDL);
}
::OleUninitialize();
return retVal;
}
How you can use my class.
+ Create two file .h and .cpp with their content as i wrote above.
+ Coppy two file into your project, add "getFolderPath.h" library in you programme
+ In main programme or when you process an event such as click to a button, you report an std::string variable to call GetFolderPath function. Example: std::string path = "";
- If your application is console application, hOwner = NULL - If it is a window application or a MFC application, you have to use it's HWND for GetFolderPath function. You can get it's HWND by this function: this->GetSafeHWND();
If success, (GetFolderPath return true) path will contain your path to folder which you choose
I use static function: static bool GetFolderPath(std::string& folderpath, HWND); so you can call it although you don't create new objectThis is example which i do with console application:
#include "getPathFolder.h"
#include <string>
int main()
{
std::string sPath = "";
if(getPathFoder::GetFolderPath(sPath,NULL))
{
printf("You have just chosen this folder: %s",sPath.c_str());
}
else
{
// Process when can get folder's path
}
return 1;
}
Best wishes for you, thanks for visiting my blog!
Do your best, the rest will come!
Convert std::string to int and vice versa
You can convert std::string to int by this syntax:
number = atoi(sNumber.c_str());
To convert int to std::string, you can convert int to char*, after that you can convert char* to std::string
To convert int to char*, you can be able to use this syntax
char str[10];
itoa(number,str,10); // 10 --> convert to 10 base
std::string sNumber = "123";
int number;
To convert int to std::string, you can convert int to char*, after that you can convert char* to std::string
To convert int to char*, you can be able to use this syntax
char str[10];
itoa(number,str,10); // 10 --> convert to 10 base
Thứ Ba, 19 tháng 7, 2011
Get all file's name and subdirectory from directory 's path
If you want to get all file's name from folder's path in C++, you can use many way. I know one way which can help you to do this work.
There are some steps you have to do:
+ The first, you must add chain: "\\*.*" after your path.
+ The second, calling FindFirstFileA function to find the first file in your folder
+ Then you calling FindNextFileA function to find all file to the end
And this is my source code that you can use:
// You have to use windows.h and tchar.h library
char * pathFolder = "D:";
sprintf(folderFind,"%s\\*.*",pathFolder);
hfind = FindFirstFileA(folderFind,&findFile);
isFinish = FindNextFileA(hfind,&findFile);
while(isFinish)
{
FileNext = findFile.cFileName; // Do with your file's name
isFinish = FindNextFileA(hfind,&findFile);
}
Best wishes for you. Thanks for visting my blog!
Do your best, the rest will come!
There are some steps you have to do:
+ The first, you must add chain: "\\*.*" after your path.
+ The second, calling FindFirstFileA function to find the first file in your folder
+ Then you calling FindNextFileA function to find all file to the end
And this is my source code that you can use:
// You have to use windows.h and tchar.h library
char * pathFolder = "D:";
char* FileNext;
WIN32_FIND_DATAA findFile;
HANDLE hfind;
char folderFind[255];
int isFinish;
HANDLE hfind;
char folderFind[255];
int isFinish;
hfind = FindFirstFileA(folderFind,&findFile);
isFinish = FindNextFileA(hfind,&findFile);
while(isFinish)
{
FileNext = findFile.cFileName; // Do with your file's name
isFinish = FindNextFileA(hfind,&findFile);
}
Best wishes for you. Thanks for visting my blog!
Do your best, the rest will come!
Convert CString to std::wstring
To convert CString to std::wstring in MFC or C++, you can use this syntax:
CString myCString = "I love Viet Nam"; // If you use Unicode format, you have to insert 'L' character before this quote
std::wstring result;
result = (LPCTSTR)outPutFolder;
We look it is same as the way that we use to convert CString to std::string when we don't use Unicode format.
Best wishes for you. Thanks for visiting my blog!
Do your best, the rest will come!
CString myCString = "I love Viet Nam"; // If you use Unicode format, you have to insert 'L' character before this quote
std::wstring result;
result = (LPCTSTR)outPutFolder;
We look it is same as the way that we use to convert CString to std::string when we don't use Unicode format.
Best wishes for you. Thanks for visiting my blog!
Do your best, the rest will come!
Chủ Nhật, 17 tháng 7, 2011
Thứ Bảy, 16 tháng 7, 2011
A good site for CString
Today i surf web and i find an good website for CString data. It has a lot of information about CString so i can't read all of it. I want to introduce to you so that we can read together and we can read faster and faster.
This is the link of the website that i found:
http://www.flounder.com/cstring.htm#Converting%20between%20char%20*%20and%20CString
I hope you will join me. Thanks a lot!
Do your best, the rest will come!
This is the link of the website that i found:
http://www.flounder.com/cstring.htm#Converting%20between%20char%20*%20and%20CString
I hope you will join me. Thanks a lot!
Do your best, the rest will come!
Convert CString to char* and vice versa in MFC
Hi everybody,I am Cuong, i come from VietNam. I have just said about some topic recently. Today i want to return "convert" topic to introduce to you how to convert CString to char* and vice versa in MFC.
Do you know about it ? And is it easy?
When i had a project some months ago, i had to use it but i didn't know and i felt that it was really difficult. But now i feel it is easy because i found one of the best way to do it.
For saving your time, i am introducing now.
CString myCString = "I love Viet Nam"; // using: L"I love Viet Nam" if you want to do with Unicode format
char myChar[1000]; // the number depends on your CString's length
//initialize your storage buffer
memset(myChar,0,sizeof(myChar));
sprintf(myChar,"%S",myCString);
Actually, you can use an easier syntax if you don't use Unicode format
myChar = myCString.GetBuffer(myCString.GetLength());
CString myCString;
char* myChar = "I love Viet Nam"; // You mustn't insert 'L' before this quote although you can use Unicode format
Maybe there are many ways to convert this type but i think this is an easy way that you can use.
Best wishes for you!, Thanks for visiting my blog!
Do your best, the rest will come!
Do you know about it ? And is it easy?
When i had a project some months ago, i had to use it but i didn't know and i felt that it was really difficult. But now i feel it is easy because i found one of the best way to do it.
For saving your time, i am introducing now.
+ Convert CString to char*
CString myCString = "I love Viet Nam"; // using: L"I love Viet Nam" if you want to do with Unicode format
char myChar[1000]; // the number depends on your CString's length
//initialize your storage buffer
memset(myChar,0,sizeof(myChar));
sprintf(myChar,"%S",myCString);
Actually, you can use an easier syntax if you don't use Unicode format
myChar = myCString.GetBuffer(myCString.GetLength());
+ Convert char* to CString
CString myCString;
char* myChar = "I love Viet Nam"; // You mustn't insert 'L' before this quote although you can use Unicode format
for(int i = 0; myChar[i] != NULL; i++)
{
myCString += myChar[i];
}Maybe there are many ways to convert this type but i think this is an easy way that you can use.
Best wishes for you!, Thanks for visiting my blog!
Do your best, the rest will come!
How can you create "Browse" button in MFC's application
Have you ever created a MFC project that you must have had "Browse" button?
How could you do it?
I have done many projects in MFC and i have some experiment about this topic.
Today i am here to say to you the way you can create a "Browse" button in MFC application.
I suppose that your application's name is DemoBrowse so there are two choice for you.
If you want to browse only one file, you can drag an button object, after that you can write it's source as:
}
If you want to get lots of files at the same time, you can use this source:
} while(pos);
}
// And now you can do anything with your paths
}
If you use Unicode format in your application, you have to insert "L" character before every quote.
Example: "*.*" --> L"*.*"
So you can create easily a "Browse" button by yourself.
Best wishes for you. Thanks for your attention!
Do your best, the rest will come!
How could you do it?
I have done many projects in MFC and i have some experiment about this topic.
Today i am here to say to you the way you can create a "Browse" button in MFC application.
I suppose that your application's name is DemoBrowse so there are two choice for you.
If you want to browse only one file, you can drag an button object, after that you can write it's source as:
void CDemoBrowseDlg::OnBnClickedButtonBrowse()
{
CFileDialog FileDialog(TRUE,
"*.*",
NULL,
OFN_HIDEREADONLY,
"All files:(*.*)|*.*| // If you want to chose all kinds of file
Files .txt (*.txt)|*.txt| // If you only want to chose .txt file
Files word (*.doc;*.docx)|*.doc;*.docx||"); // If you want to chose .doc and .docx file
if (FileDialog.DoModal() == IDOK)
{
CString pathName = FileDialog.GetPathName();
// And now you can do anything with this path
}
If you want to get lots of files at the same time, you can use this source:
void CDemoBrowseDlg::OnBnClickedButtonBrowse()
{
int i = 0;
{
int i = 0;
CString arrayListFile[100]; // CString array which contains paths
UpdateData(TRUE);
CFileDialog FileDialog(TRUE,
UpdateData(TRUE);
CFileDialog FileDialog(TRUE,
"*.*",NULL,OFN_HIDEREADONLY | OFN_ALLOWMULTISELECT,
"Image Files (*.jpg;*.bmp;*.png;*.gif;*.ico;*.pbm;*.tif;*.pgm;*.xpm)|
"Image Files (*.jpg;*.bmp;*.png;*.gif;*.ico;*.pbm;*.tif;*.pgm;*.xpm)|
*.jpg;*.bmp;*.png;*.gif;*.ico;*.pbm;*.tif;*.pgm;*.xpm|
Files(*.txt;*.docx)|*.txt;*.docx||");
// Create buffer for ten filenames
TCHAR strFiles[MAX_PATH * 100] = "";
FileDialog.m_ofn.lpstrFile = strFiles;
FileDialog.m_ofn.nMaxFile = sizeof(strFiles);
// Create buffer for ten filenames
TCHAR strFiles[MAX_PATH * 100] = "";
FileDialog.m_ofn.lpstrFile = strFiles;
FileDialog.m_ofn.nMaxFile = sizeof(strFiles);
// If it has err here, you can use a number ( > 0) to replace sizeof(strFiles). I haven't also known why it has err
if(FileDialog.DoModal() == IDOK)
{
POSITION pos = FileDialog.GetStartPosition();
if(pos)
{
CString PathName;
do
{
PathName = FileDialog.GetNextPathName(pos);
arrayListFile[i] = PathName;
i++;if(FileDialog.DoModal() == IDOK)
{
POSITION pos = FileDialog.GetStartPosition();
if(pos)
{
CString PathName;
do
{
PathName = FileDialog.GetNextPathName(pos);
arrayListFile[i] = PathName;
} while(pos);
}
// And now you can do anything with your paths
}
If you use Unicode format in your application, you have to insert "L" character before every quote.
Example: "*.*" --> L"*.*"
So you can create easily a "Browse" button by yourself.
Best wishes for you. Thanks for your attention!
Do your best, the rest will come!
Thứ Tư, 13 tháng 7, 2011
Convert CString to std::string and vice versa in MFC
Hi all,
Today i return my blog and i want to be able to tell about converting kinds of data.
Do you know how to convert between CString and std::string?
This is very important for progamming with MFC application.
There are many ways to do this but i will introduce one of them.
If you want to convert from std::string to CString, you can use syntax:
And if you want to convert vice versa, you can do the following:
I hope you will succeed. Thanks for visiting my blog!
Do your best, the rest will come.
Today i return my blog and i want to be able to tell about converting kinds of data.
Do you know how to convert between CString and std::string?
This is very important for progamming with MFC application.
There are many ways to do this but i will introduce one of them.
If you want to convert from std::string to CString, you can use syntax:
std::string demo("You are welcome!");
CString convert;
convert(demo.c_str());And if you want to convert vice versa, you can do the following:
CString demo("You are welcome!");
std::string convert;
// If you don't use Unicode format
convert((LPCTSTR)demo);
// If you use Unicode format
CT2CA temp(demo);
convert(temp);I hope you will succeed. Thanks for visiting my blog!
Do your best, the rest will come.
Thứ Hai, 11 tháng 7, 2011
Convert CString to int and vice versa in MFC
Today, i introduce you some ways to convert in MFC application. They will help you when you programme with MFC application.
We will begin with CString and int formats.
If you want to convert from int number to CString you can use syntax:
You will be able to convert CString to int if you use that syntax:
I hope it will help you. Please email to me if you need some help.
Thanks for visiting my blog!
We will begin with CString and int formats.
If you want to convert from int number to CString you can use syntax:
int numberNeedConvert;
CString numberConvert;
...
// If you do not use unicode format for your progamme numberConvert.Format("%d",numberNeedConvert);
// If you use unicode format for your programme
numberConvert.Format(L"%d",numberNeedConvert); You will be able to convert CString to int if you use that syntax:
int number;
CString cstringNeedConvert;
char* temp;
...
// Convert CString to char* here
char* temp;
...
// Convert CString to char* here
...
number = atoi(temp);
I hope it will help you. Please email to me if you need some help.
Thanks for visiting my blog!
Thứ Năm, 23 tháng 6, 2011
UDD path for Olly Debug
Today, i do research about "Config your UDD folder's path on Olly debug". Something about my articles:
+ A UDD path is the path on your hard drive to the folder where OllyDbg stores its UDD files.
+ UDD files are files OllyDbg uses to store information it gathers about a process with its analyzer.
So, We can understand that UDD is a part of Olly Debug that Olly Debug need it to load path on your hard drive to the folder where OllyDbg stores its UDD files, if you do not config this path, you will get some problems when you debug your application.
UDD files use to store information it gathers about a process with its analyzer.
Thus, I think that UDD path does not affect to Debug Process.
Thứ Tư, 22 tháng 6, 2011
BNF for fuzzing
I am researching about BNF. I am trying to make an application that check many files' formats. I have done in .pls format for Winamp, MediaMonkey and .m3u format for VLC and MediaMonkey. I found some problem in these applications. If we use a very long file's name to definite a playlist (file .pls or .m3u), Winamp or Mediamonkey will be crash.
Now i am trying to discover this error, it can be use to attack some systems.
Now i am trying to discover this error, it can be use to attack some systems.
Thứ Ba, 21 tháng 6, 2011
Đăng ký:
Bài đăng (Atom)
Install Kubernetes using kubeadm with two nodes master & worker
Well come back for a long time my friends! Today I want to take a note about some basic knowledge about Kubernetes. I hope that it will...
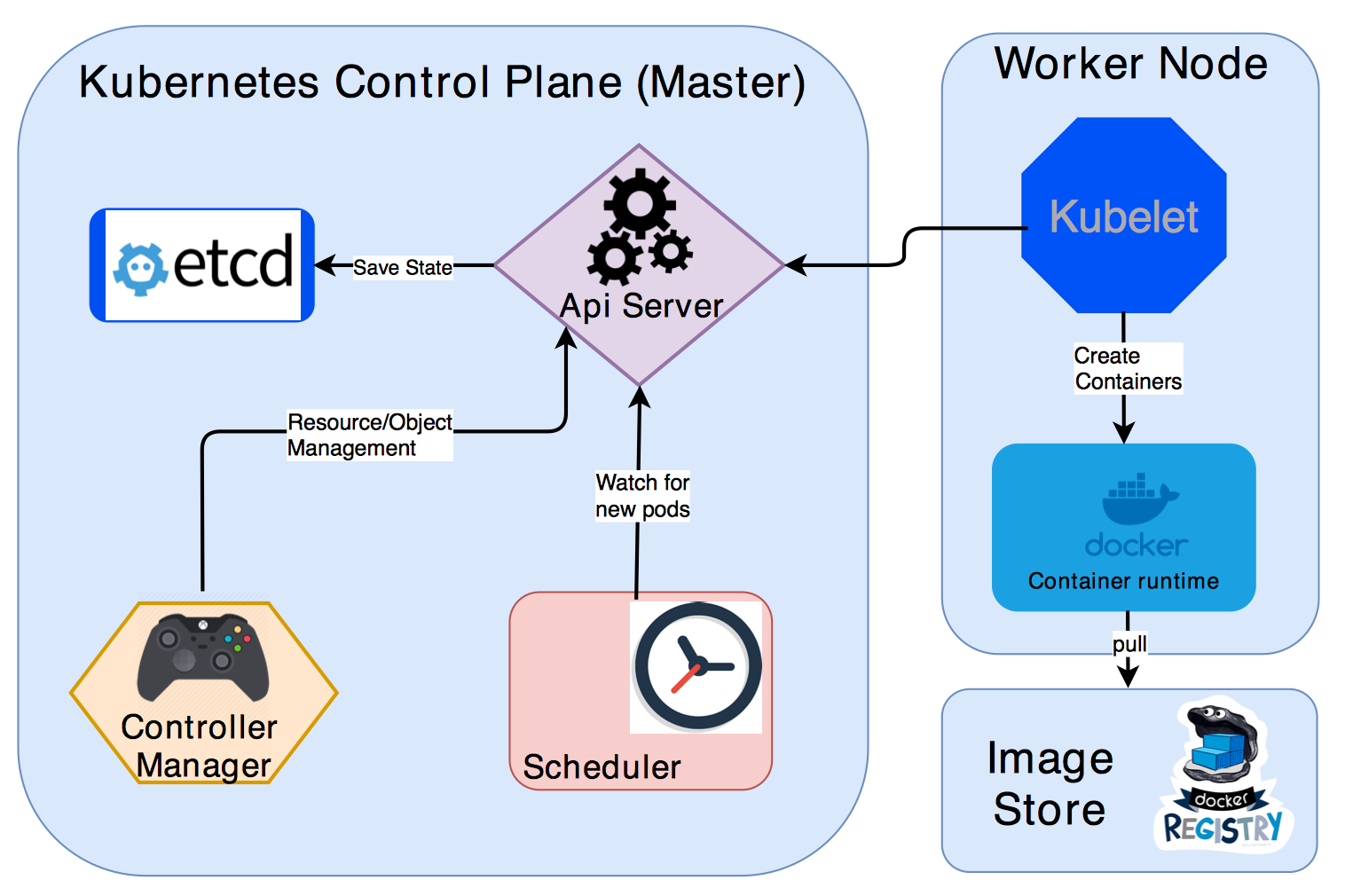
-
Today, i introduce you some ways to convert in MFC application. They will help you when you programme with MFC application. We will begin...
-
Well come back for a long time my friends! Today I want to take a note about some basic knowledge about Kubernetes. I hope that it will...
-
To convert CString to std::wstring in MFC or C++, you can use this syntax: CString myCString = "I love Viet Nam"; // If ...